공모일정정 및 신규 상장일일 가져오기위해서 38.co.kr에서 데이터를 가져 오는 부분입니다.
tdm1, slack 부분은 개인적으로 사용하는 공통부분입니다.
개발에 참고하세요~
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
|
import requests
from bs4 import BeautifulSoup
from Tdm1 import *
from ChartStock import *
import datetime
from slack import *
class Site38():
def __init__(self, tdsql):
self.tdsql = tdsql
self.tdm1 = Tdm1(tdsql)
def main(self):
self.getNewStock()
self.getNewOpenStock()
def checkmain(self):
self.checkTodayNewStock()
self.checkTodayNewOpenStock()
def getNewOpenStock(self):
_url = 'https://www.38.co.kr/html/fund/index.htm?o=nw'
response = requests.get(_url)
response.raise_for_status()
html = response.text
soup = BeautifulSoup(html, 'html.parser')
for ii in range(1, 21):
sii = str(ii)
_title = soup.select_one('body > table:nth-child(9) tbody > tr:nth-child(' + sii + ') > td:nth-child(1)').get_text()
_title = _title.replace('(유가)', '')
_title = _title.strip()
_dt = soup.select_one('body > table:nth-child(9) tbody > tr:nth-child(' + sii + ') > td:nth-child(2)').get_text()
_dt = _dt.replace('/', '')
_dt = _dt.strip()
if(len(self.tdm1.getStack('NEWOPEN',_title)) < 1):
self.tdm1.setStack('NEWOPEN', _title, _dt, '')
def getNewStock(self):
_url = 'http://www.38.co.kr/html/fund/index.htm?o=k'
response = requests.get(_url)
response.raise_for_status()
html = response.text
soup = BeautifulSoup(html, 'html.parser')
for ii in range(1,26):
sii = str(ii)
_title = soup.select_one('body > table:nth-child(9) tbody > tr:nth-child('+sii+') > td:nth-child(1)').get_text()
_title = _title.replace('(유가)','')
_title = _title.strip()
_dt = soup.select_one('body > table:nth-child(9) tbody > tr:nth-child(' + sii + ') > td:nth-child(2)').get_text()
_dt = _dt.split('~')[0].replace('.','')
_dt = _dt.strip()
_price = soup.select_one('body > table:nth-child(9) tbody > tr:nth-child(' + sii + ') > td:nth-child(3)').get_text()
_price = _price.split('~')[0].replace('.', '')
_price = _price.strip()
_cnt = self.tdm1.getList1('new_stock_cnt',_title)['cnt'][0]
if _cnt == 0:
self.tdm1.excute3('new_stock_intsert',_title, _dt, _price)
else:
self.tdm1.excute3('new_stock_update', _dt, _price, _title)
def checkTodayNewStock(self):
now = datetime.datetime.now()
nowDate = now.strftime('%Y%m%d')
df = self.tdm1.getList1('new_stock_today_chck',nowDate)
_size = len(df.index)
if _size >0 :
for index, row in df.iterrows():
slack().send('공모주청약:'+row['name']+':'+row['dt']+':'+row['amt'])
def checkTodayNewOpenStock(self):
now = datetime.datetime.now()
nowDate = now.strftime('%Y%m%d')
df = self.tdm1.getStack2('NEWOPEN',nowDate)
_size = len(df.index)
if _size >0 :
for index, row in df.iterrows():
slack().send('오늘상장:'+row['COL1'])
if __name__ == '__main__':
nv = Site38(None)
nv.main()
nv.checkmain()
|
cs |
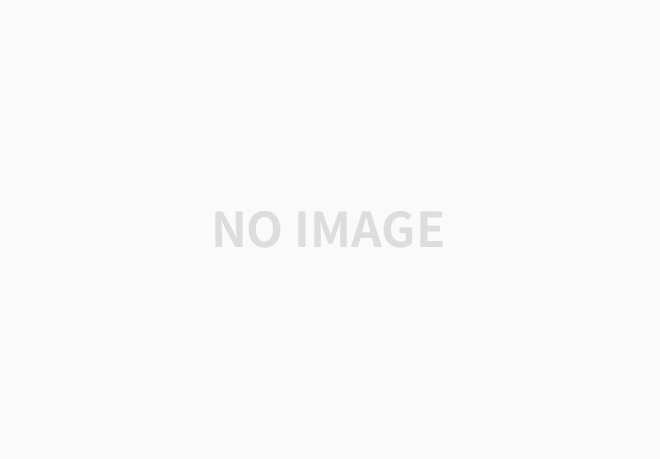